APIs (Application Programming Interfaces) are the foundation of modern applications, enabling seamless communication between systems, services, and devices. Whether designing a RESTful API or leveraging GraphQL, following best practices ensures efficiency, security, and scalability. In this blog, we’ll explore essential API design and integration principles to help you build high-performing APIs.
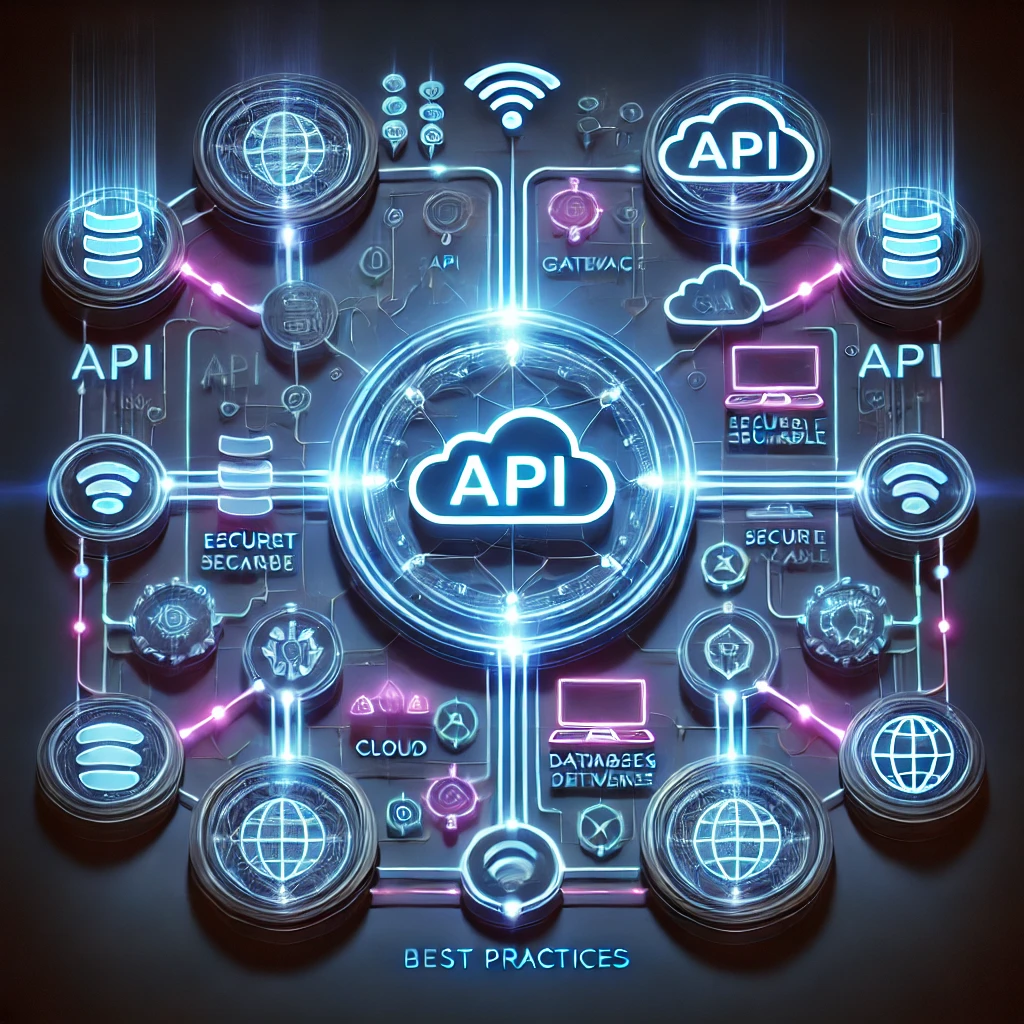
1. Select the Proper API Architecture
Choosing the correct API architecture is crucial for performance and usability. The most widely used architectures are:
- REST (Representational State Transfer): A stateless architecture employing standard HTTP methods, most widely used for web services.
- GraphQL: A query language for APIs that allows clients to specify the exact data they need, minimizing over-fetching and under-fetching.
Choose REST if you need simplicity and widespread support. Opt for GraphQL when dealing with complex data relationships and dynamic front-end applications. If you’re building a microservices ecosystem, consider gRPC, which offers high-performance, low-latency communication.
2. Keep API Endpoints Intuitive and Consistent
- Use clear, predictable URLs (e.g., /users/{id} instead of /getUser?id=123).
- Follow RESTful conventions (e.g., GET for reading, POST for creating, PUT/PATCH for updating, and DELETE for removing resources).
- Avoid unnecessary nesting; deep hierarchical URLs can complicate API usage.
- Keep endpoints resource-oriented and avoid action-based naming (e.g., /updateUser should be /users/{id} with the appropriate HTTP method).
3. Optimize Performance with Pagination and Caching
To prevent performance bottlenecks:
- Implement Pagination: Return only a subset of data using limit/offset or cursor-based pagination (e.g., ?limit=10&offset=20).
- Use Caching: Reduce redundant requests with HTTP caching (ETags, Last-Modified headers) or in-memory caching with Redis.
- Optimize Database Queries: Utilize indexing and prevent N+1 query issues in SQL databases.
- Enable Compression: Minimize payload size with GZIP compression to enhance response times.
4. Prioritize Security Measures
Security is non-negotiable in API development. Implement the following:
- Authentication & Authorization: Secure endpoints using OAuth 2.0, JWT (JSON Web Tokens), or API keys.
- Rate Limiting: Thwart abuse with rate limits (e.g., X-Rate-Limit headers).
- Data Encryption: Utilize HTTPS and encrypt sensitive information.
- Input Validation: Prevent SQL injection and XSS attacks by sanitizing inputs.
- Use Secure Headers: Implement security headers like Content-Security-Policy and Strict-Transport-Security to mitigate vulnerabilities.
5. Use Proper Status Codes and Error Handling
- Return appropriate HTTP status codes (200 OK, 201 Created, 400 Bad Request, 404 Not Found, 500 Internal Server Error).
- Provide detailed error messages with meaningful descriptions and error codes.
- Implement global error handling to ensure consistency.
- Structure error responses using a standard format, such as:
{
“error”: {
“code”: 400,
“message”: “Invalid request parameters”
}
}
6. Ensure API Versioning
APIs evolve, and breaking changes can disrupt clients. Implement versioning strategies like:
- URL versioning: /v1/users
- Header-based versioning: Accept: application/vnd.myapi.v1+json
- Query parameter versioning: ?version=1
- Deprecate old versions gradually while ensuring proper communication with API consumers.
7. Write Comprehensive API Documentation
Clear documentation improves API adoption and usability. Use tools like Swagger (OpenAPI), Postman, or GraphQL Playground to:
- Provide request/response examples.
- List all available endpoints, parameters, and expected behaviors.
- Include authentication details and error codes.
- Offer a sandbox environment for developers to test API requests.
8. Implement Webhooks and Real-Time Capabilities
For real-time applications, consider:
- WebSockets: Persistent bidirectional communication (e.g., chat apps, live notifications).
- Webhooks: Event-driven API communication (e.g., notifying external systems of updates).
- Server-Sent Events (SSE): Push notifications over HTTP without requiring WebSockets.
- Message Queues: Use services like Kafka or RabbitMQ to handle asynchronous background tasks efficiently.
9. Focus on API Testing and Monitoring
Ensure reliability by:
- Automated Testing: Use tools like Postman, Jest, or Mocha for unit and integration testing.
- Monitoring & Logging: Track API health using Prometheus, New Relic, or API Gateway logs.
- Error Tracking: Identify issues with tools like Sentry or LogRocket.
- Performance Testing: Use JMeter or k6 to evaluate API load handling.
10. Design for Scalability
APIs must handle increasing traffic and evolving business needs. Enhance scalability with:
- Load Balancing: It Distributes the traffic across multiple servers to prevent from overload.
- Microservices Architecture: Decouple services for flexibility and independent scaling.
- Asynchronous Processing: Use message queues like RabbitMQ or Kafka to manage background tasks efficiently.
- API Gateways: Implement gateways such as Kong or AWS API Gateway to handle routing, authentication, and rate-limiting.
- Horizontal Scaling: Architect APIs to handle increased loads by deploying across multiple servers or cloud instances.
Conclusion
Following these best practices in API design and integration enhances efficiency, security, and scalability. Whether building RESTful or GraphQL APIs, prioritizing clear design, security, and performance will lead to robust and maintainable applications. Additionally, continuously monitoring API usage and iterating based on analytics will help ensure long-term success. By implementing these principles, you’ll create APIs that provide seamless user experiences and long-term reliability.